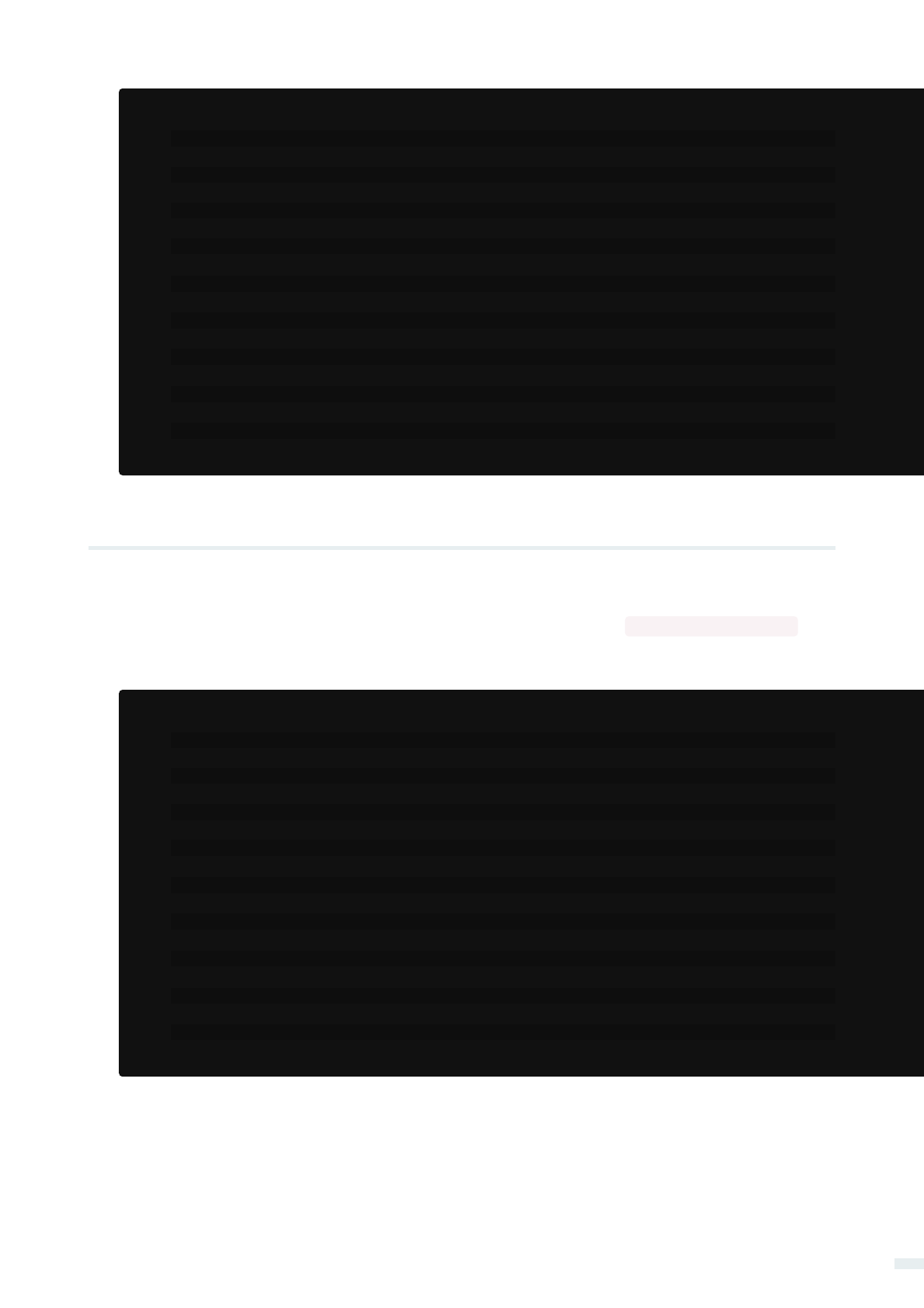
The above code loops through each row in the spreadsheet and grabs the cells as a list. Each validates
method checks the value of a cell and returns an error message if the value is invalid.
This code creates a new spreadsheet, specifies headers, and outputs the error message results so that there
is a log of invalid data.
1.
2.
3.
4.
5.
6.
7.
8.
9.
10.
11.
12.
13.
14.
15.
16.
17.
18.
19.
'Iterate through the rows
For i = 2 To 101
Dim result = New PersonValidationResult With {.Row = i}
results.Add(result)
'Get all cells for the person
Dim cells = worksheet($"A{i}:E{i}").ToList()
'Validate the phone number (1 = B)
Dim phoneNumber = cells(1).Value
result.PhoneNumberErrorMessage = ValidatePhoneNumber(phoneNumberUtil, CStr(phoneNumber))
'Validate the email address (3 = D)
result.EmailErrorMessage = ValidateEmailAddress(CStr(cells(3).Value))
'Get the raw date in the format of Month Day[suffix], Year (4 = E)
Dim rawDate = CStr(cells(4).Value)
result.DateErrorMessage = ValidateDate(rawDate)
Next i
Use IronXL to validate a spreadsheet of data. The DataValidation sample uses to
validate phone numbers and uses standard C# APIs to validate email addresses and dates.
7. Validate Spreadsheet Data
libphonenumber-csharp
Sample: DataValidation
1.
2.
3.
4.
5.
6.
7.
8.
9.
10.
11.
12.
13.
14.
15.
16.
17.
18.
19.
'Iterate through the rows
For y = 2 To 101
Dim result = New PersonValidationResult With {.Row = y}
results.Add(result)
'Get all cells for the person
Dim cells = worksheet($"A{y}:E{y}").ToList()
'Validate the phone number (1 = B)
Dim phoneNumber = cells(1).Value
result.PhoneNumberErrorMessage = ValidatePhoneNumber(phoneNumberUtil, CStr(phoneNumber))
'Validate the email address (3 = D)
result.EmailErrorMessage = ValidateEmailAddress(CStr(cells(3).Value))
'Get the raw date in the format of Month Day[suffix], Year (4 = E)
Dim rawDate = CStr(cells(4).Value)
result.DateErrorMessage = ValidateDate(rawDate)
Next y
6